2023年7月4日-孙昱霖-工作日志
点击数:111
一·工作内容
1·ChatGPT使用,问答,游戏编写
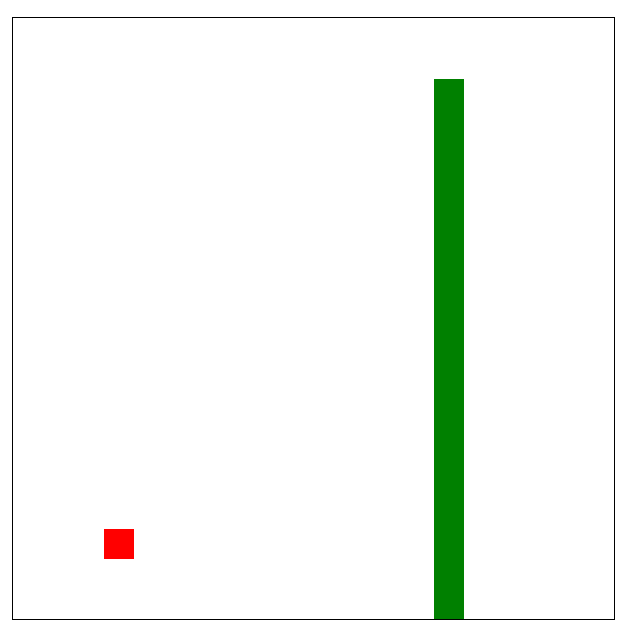
代码如下:
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇游戏</title>
<style>
#game-board {
width: 400px;
height: 400px;
border: 1px solid black;
}
.cell {
width: 20px;
height: 20px;
float: left;
}
.snake {
background-color: green;
}
.food {
background-color: red;
}
</style>
</head>
<body>
<h1>贪吃蛇游戏</h1>
<div id="game-board"></div>
<script>
var gameBoard = document.getElementById("game-board");
var gridSize = 20;
var snake = [{ x: 10, y: 10 }];
var food = { x: 5, y: 5 };
var direction = "right";
var gameInterval;
function drawGameBoard() {
var cells = "";
for (var i = 0; i < gridSize; i++) {
for (var j = 0; j < gridSize; j++) {
var cellClass = "cell";
if (isSnakeCell(j, i)) {
cellClass += " snake";
} else if (isFoodCell(j, i)) {
cellClass += " food";
}
cells += "<div class='" + cellClass + "'></div>";
}
}
gameBoard.innerHTML = cells;
}
function isSnakeCell(x, y) {
for (var i = 0; i < snake.length; i++) {
if (snake[i].x === x && snake[i].y === y) {
return true;
}
}
return false;
}
function isFoodCell(x, y) {
return food.x === x && food.y === y;
}
function handleKeydown(event) {
if (event.keyCode === 37 && direction !== "right") {
direction = "left";
} else if (event.keyCode === 38 && direction !== "down") {
direction = "up";
} else if (event.keyCode === 39 && direction !== "left") {
direction = "right";
} else if (event.keyCode === 40 && direction !== "up") {
direction = "down";
}
}
function updateGame() {
var head = Object.assign({}, snake[0]);
if (direction === "left") {
head.x--;
} else if (direction === "up") {
head.y--;
} else if (direction === "right") {
head.x++;
} else if (direction === "down") {
head.y++;
}
snake.unshift(head);
if (isFoodCell(head.x, head.y)) {
food.x = Math.floor(Math.random() * gridSize);
food.y = Math.floor(Math.random() * gridSize);
} else {
snake.pop();
}
drawGameBoard();
}
function startGame() {
clearInterval(gameInterval);
snake = [{ x: 10, y: 10 }];
direction = "right";
gameInterval = setInterval(updateGame, 200);
}
document.addEventListener("keydown", handleKeydown);
startGame();
</script>
</body>
</html>
2·安装了2自由度舵机
3·学习了ESP8266 D1 mini主板,控制LED灯闪烁,控制点头摇头
4·制作了机器人的主体结构
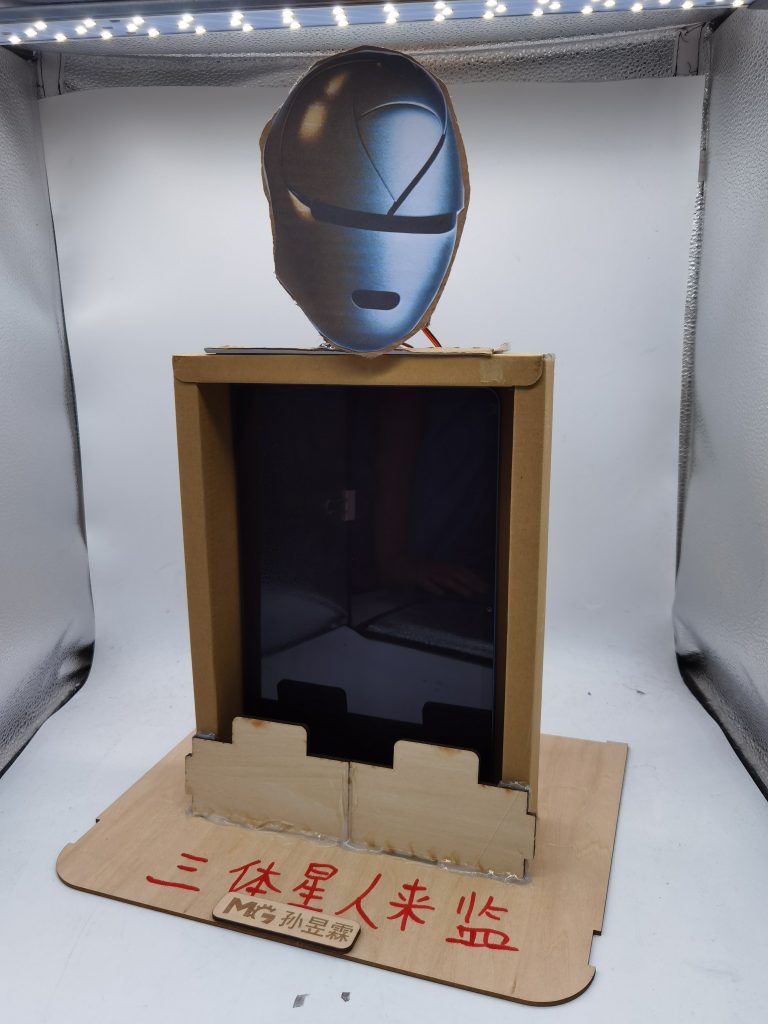
二·感悟